How to script ChatGPT in Excel with VBA
Learn to combine GPT for Excel and VBA to create workflows around AI actions (such as content generations, translations or classifications) inside Excel:
- With the GPT for Excel add-in, you can use ChatGPT and other AIs in Excel through custom functions such as GPT(), GPT_TRANSLATE(), GPT_CLASSIFY(), which you can type inside cells.
- With VBA, you can automate the creation of these functions and manipulate their results.
Here are some scenarios where integrating ChatGPT in Excel with VBA can be particularly useful:
- Automating repetitive tasks across multiple worksheets: Generate company profiles, descriptions, or industry insights with ChatGPT and automate their application to other companies across worksheets using VBA.
- Validating outputs: Use ChatGPT to edit content, translate text, or summarize reports, then validate the output with VBA.
- Integrating Excel with other Microsoft tools: Use VBA to automate sending emails or exporting data.
Here are the 3 steps to get started combining GPT for Excel with VBA:
- Install the GPT for Excel add-in.
- Write a GPT formula to ensure it works.
- Manipulate GPT functions with an Excel macro using VBA
The following sections will walk you through each of these steps in detail.
Step #1: Install GPT for Excel add-on
To install GPT for Excel add-in, follow these steps:
- Visit the GPT for Excel Word addin-in installation page.
- Click Open in Excel to start the integration process.
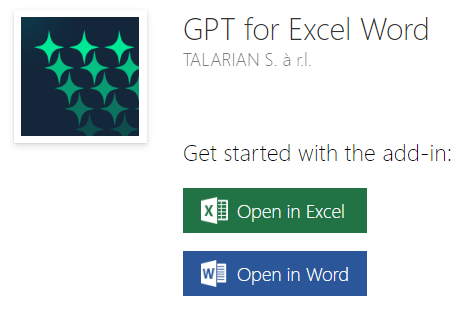
- Confirm any permissions to proceed with installing the Excel GPT extension.
- To open the sidebar, click GPT for Excel Word in the Home tab. If you don't see it, go to Home > Add-ins > My add-ins > GPT for Excel Word.
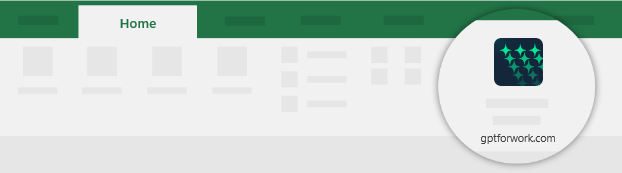
Chat GPT is now connected to Microsoft Excel and can be used directly from any of your workbooks. Available models include GPT-4o, GPT-4o mini, o1, OpenAI Assistants, Claude 3.5 Sonnet and many more.
.png?table=block&id=1997455f-b966-80da-937b-ed3face271f3&cache=v2)
The installation details are also outlined in the following ChatGPT for Excel guide page.
Step #2: Test the ChatGPT integration in Excel
The GPT for Excel add-in offers several functions:
- GPT(): The simplest function for prompting in Excel cells
- GPT_TRANSLATE(): A specific function for translation
- GPT_CLASSIFY(): Another function for text content classification
- ...
You can find the complete list of available functions on this page.
To integrate GPT for Excel formulas with VBA, we’ll use a simple example featuring the GPT() function. The same process applies to the other functions.
Follow these steps:
- Open a new Excel file
- Save the file with the .xlsm extension to enable macros
.jpg?table=block&id=1997455f-b966-807c-99b7-f216e6f98516&cache=v2)
- Open your saved Excel file.
- Launch the GPT for Excel extension.
- In Sheet1, type the following formula into cell A1:
=GPT("hello")
You should see a response generated by GPT in cell A1, such as:
.png?table=block&id=1997455f-b966-8083-be07-f8c0bd7b3382&cache=v2)
You have now successfully used a GPT function inside Excel.
Now, let’s say you need to trigger this function through VBA. This will be the focus of the next section: "Connect your GPT function in VBA."
Step #3: Prompt ChatGPT with an Excel macro using VBA
Simple insertion of a GPT formula
- Click on the “Developer” tab of your Excel file (if you don’t see this tab, refer to the instructions on this Microsoft page to enable it).
.jpg?table=block&id=1997455f-b966-809d-9436-c9652ffba9e5&cache=v2)
- Click on Visual basic in the Developer tab to open the VBA editor
.jpg?table=block&id=1997455f-b966-8080-acf1-fa771336dafb&cache=v2)
- On the left side of the screen in the Project Explorer, right-click on 'Sheet1' and select Insert > Module
.png?table=block&id=1997455f-b966-8082-af23-ee01b6649ff7&cache=v2)
- In the blank module screen that appears, type the following code:
Sub WriteGPTFormula()
'Insert the GPT function into cell A2 of Sheet1
Sheets("Sheet1").Range("A2").Formula = "=GPT(""Hello"")"
End Sub
- Click on the Save button to save your VBA code.
- Then, click the Run Sub/UserForm button (green triangle) in the toolbar to execute the macro.
.jpg?table=block&id=1997455f-b966-8017-9137-c69303740c29&cache=v2)
You should see another response to your "Hello" in cell A2, such as:
.jpg?table=block&id=1997455f-b966-80a7-a0fd-f118f5193c20&cache=v2)
If you prefer referring to cells instead of ranges, you can alternatively use the following code:
Sub WriteGPTFormula()
Sheets("Sheet1").Cells(2, 1).FormulaLocal = "=GPT(" & """Hello""" & ")"
End Sub
This will give you the same result as the first code.
Using the result of a GPT function in VBA
Initial test
Now, let’s say you want to save the result of the GPT("Hello") prompt and display it in a MsgBox. You might write code like this:
Sub WriteGPTFormula()
Sheets("Sheet1").Cells(1, 1).FormulaLocal = "=GPT(" & """Hello""" & ")"
X = Sheets("Sheet1").Cells(1, 1).Value
MsgBox X
End Sub
However, you will encounter an error message: "Subscript out of range". This happens because the GPT function needs time to process the prompt, but the ongoing VBA code doesn’t wait for it to complete, causing a conflict.
.png?table=block&id=1997455f-b966-80ae-b1d6-d72d856d3801&cache=v2)
Trying to bypass this issue by combining Application.CalculationState and DoEvents will not resolve the problem. The A1 cell will continue to display a #BUSY! message. For example:
Sub WriteGPTFormula()
Sheets("Sheet1").Cells(1, 1).FormulaLocal = "=GPT(" & """Hello""" & ")"
Do While Application.CalculationState <> xlDone
DoEvents
Loop
X = Sheets("Sheet1").Cells(1, 1).Value
MsgBox X
End Sub
The issue here is that the GPT() function cannot complete its execution as long as DoEvents or Application.Calculate is actively running.
Solution
To resolve this issue, we need to give the GPT() function time to execute without blocking it. For instance, we can wait for 3 to 5 seconds before checking if the function has completed. If the function has finished, we can retrieve the result. Otherwise, we schedule another check.
This requires two separate VBA procedures:
1. Sub WriteGPTFormula() - Purpose: Initiates the process by inserting the =GPT(“hello”) formula into cell A1 and schedules the first check to retrieve the result.
2. Sub CheckGPTResult() - Purpose: Checks if the function GPT() has completed and retrieves the result from cell A1. If the result is not yet available, it reschedules itself to check again after a delay
Full code:
//
Sub WriteGPTFormula()
' Write the formula =GPT(“Hello”) in cell A1
Sheets("Sheet1").Cells(1, 1).FormulaLocal = "=GPT(" & """Hello""" & ")"
' Schedule the first check in 5 seconds
Application.OnTime Now + TimeValue("00:00:03"), "CheckGPTResult"
End Sub
Sub CheckGPTResult()
Dim X As Variant
Dim NextCheck As Date
' Retrieve the value from cell A1
X = Sheets("Sheet1").Range("A1").Value
' Check if the cell contains an error or is empty
If IsError(X) Or X = "" Then
' If the cell is still calculating or empty, schedule another check
NextCheck = Now + TimeValue("00:00:03") ' Check again in 5 seconds
Application.OnTime NextCheck, "CheckGPTResult"
Else
' The cell contains a value, display the result
MsgBox X
End If
End Sub
//
Conclusion
In conclusion, integrating Chat GPT for Excel with VBA opens up powerful possibilities for automating advanced and repetitive tasks in Excel. By combining the flexibility of VBA with the AI-driven capabilities of ChatGPT, you can create dynamic workflows that go beyond standard Excel functionalities. Whether it's applying consistent formulas across multiple sheets, leveraging AI outputs for advanced operations, or connecting Excel with other Microsoft tools, this integration will help you work way faster.
The step-by-step guide provided demonstrates how to install the GPT for Excel add-in, test its functionality, and use VBA to trigger GPT formulas, handle their outputs, and manage execution delays effectively. By following these instructions, users can incorporate AI into their Excel macros and unlock new levels of efficiency in data management and analysis.